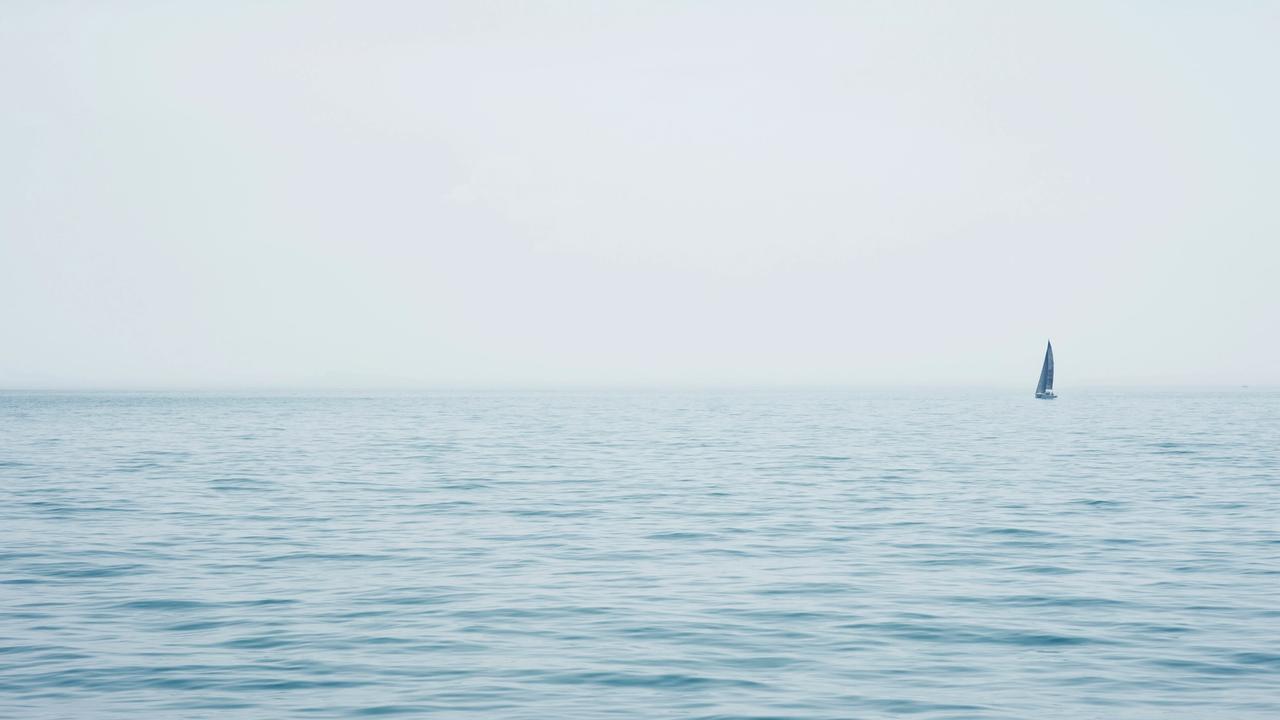
Understanding useEffect, useCallback, and useMemo in React

React and Next.js provide several hooks for managing state and optimizing component behavior. Among these, useEffect
, useCallback
, and useMemo
are essential for handling side effects, memoizing functions, and optimizing rendering performance. Understanding how and when to use these hooks effectively can significantly enhance our application's efficiency.
useEffect
The useEffect
hook is used in React to perform side effects in functional components. Side effects are operations that affect state or interact with the outside world (like data fetching, updating the DOM, or subscribing to events). The hook runs after the render process, ensuring the component's output is in sync with any updates.
Usage:
jsx
useEffect(() => { // Code to run after every render console.log('Component rendered or updated'); // Optional cleanup function return () => { console.log('Component unmounted or updated'); }; }, [dependency]);
- Dependencies:
useEffect
takes an optional dependency array as its second argument. If provided, the effect runs only when the specified dependencies change. If the array is empty ([]
), the effect runs once when the component mounts. Omitting the dependency array causes the effect to run on every render. - Cleanup Function: The function returned from the effect can be used to clean up resources or subscriptions.
Example:
Fetching data from an API on component mount:
jsx
import { useEffect, useState } from 'react'; function Component() { const [data, setData] = useState(null); useEffect(() => { const fetchData = async () => { const response = await fetch('/api/data'); const result = await response.json(); setData(result); }; fetchData(); }, []); // Empty array means it runs only once return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>; }
Typical Use Cases:
- Data Fetching: Fetch data from an API when the component mounts.
- Event Listeners: Add event listeners (e.g., window resize or scroll events) when the component is rendered, and clean them up when the component is unmounted.
- Subscriptions: Subscribe to a WebSocket or other real-time data sources, and clean up the subscription when the component unmounts.
Best Practices:
- Always specify dependencies in the dependency array to avoid unwanted re-runs.
- Use the cleanup function to avoid memory leaks when dealing with subscriptions or event listeners.
- Avoid placing complex logic or functions directly in the
useEffect
body. Instead, define them outside and call them inside the effect.
useCallback
The useCallback
hook memoizes a function, ensuring that it maintains the same reference between renders unless its dependencies change. This helps to optimize the rendering behavior, particularly when passing functions as props to child components that might unnecessarily re-render otherwise.
Usage:
jsx
const memoizedCallback = useCallback(() => { // Function logic here }, [dependency]);
- Dependencies: If any of the dependencies change, the function reference is updated. If the dependencies remain the same, the cached version of the function is reused.
Example:
Using useCallback
to prevent unnecessary re-renders:
jsx
import { useCallback, useState } from 'react'; function ParentComponent() { const [count, setCount] = useState(0); const handleClick = useCallback(() => { console.log('Button clicked'); }, []); // Function is recreated only when the dependencies change return ( <div> <button onClick={handleClick}>Click me</button> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}> Increment </button> </div> ); }
Here, handleClick
maintains the same reference across renders, avoiding unnecessary re-renders of child components that receive it as a prop.
Typical Use Cases:
- Passing Callback Functions to Child Components: If pass a function as a prop to a child component and want to avoid re-rendering the child unless the function’s logic actually changes,
useCallback
is useful. - Event Handlers: Memoize event handler functions to avoid creating new function references on every render.
- Dependencies for
useEffect
: When used in conjunction withuseEffect
,useCallback
can help to avoid triggering effects unnecessarily by ensuring function dependencies are stable.
Best Practices:
- Use
useCallback
only when specific performance issue or anticipate one. Overusing it can actually degrade performance if not necessary. - Be cautious when specifying dependencies in the dependency array, ensuring all relevant variables are included.
useMemo
The useMemo
hook memoizes the result of a calculation and returns the cached result until its dependencies change. This is useful for expensive computations or rendering optimizations.
Usage:
jsx
const memoizedValue = useMemo(() => { // Calculation logic here return someValue; }, [dependency]);
Example:
Optimizing an expensive calculation:
jsx
import { useMemo, useState } from 'react'; function ExpensiveComponent() { const [count, setCount] = useState(0); const expensiveCalculation = useMemo(() => { console.log('Running expensive calculation'); return count * 2; }, [count]); // Recomputed only when count changes return ( <div> <p>Expensive Calculation: {expensiveCalculation}</p> <button onClick={() => setCount(count + 1)}> Increment </button> </div> ); }
In this case, expensiveCalculation
runs only when count
changes, avoiding unnecessary calculations.
Typical Use Cases:
- Expensive Computations: Memoize the result of an expensive calculation (e.g., sorting or filtering large data sets) to avoid recomputing it on every render.
- Optimizing Component Rendering: When rendering complex JSX trees or data transformations,
useMemo
can prevent unnecessary recalculations. - Memoizing Derived State: If derive a piece of state from other state values and the calculation is expensive,
useMemo
ensures the derived state is recalculated only when needed.
Best Practices:
- Use
useMemo
for computationally expensive operations where performance gains are noticeable. - Avoid wrapping simple calculations in
useMemo
, as this adds unnecessary complexity and overhead. - Make sure the dependencies are set correctly; if miss a dependency, the memoized value may become stale.
When to Use Each Hook
useEffect
: For side effects such as data fetching, subscriptions, or updating the DOM after rendering.useCallback
: For memoizing functions to prevent unnecessary re-renders, especially when passing callbacks to child components.useMemo
: For memoizing the result of an expensive calculation to optimize rendering.
Together, these hooks allow for more efficient and reactive components in React and Next.js applications.
How These Hooks Work Together
These hooks can complement each other to build a highly optimized React or Next.js application. Here's an example scenario that demonstrates how they might work together:
Example Scenario
Imagine building a component that displays a list of users fetched from an API, allowing for sorting and filtering, with the ability to select items from the list. Here's how can apply these hooks:
jsx
import { useEffect, useState, useCallback, useMemo } from 'react'; function UserList() { const [users, setUsers] = useState([]); const [filter, setFilter] = useState(''); const [selectedUsers, setSelectedUsers] = useState([]); // Fetch users data when the component mounts useEffect(() => { const fetchData = async () => { const response = await fetch('/api/users'); const data = await response.json(); setUsers(data); }; fetchData(); }, []); // Empty dependency array means it runs once on mount // Filtered users based on search criteria, using useMemo for performance optimization const filteredUsers = useMemo(() => { return users.filter(user => user.name.toLowerCase().includes(filter.toLowerCase())); }, [users, filter]); // Recalculate only when users or filter changes // Function to handle user selection, memoized using useCallback const toggleUserSelection = useCallback( (userId) => { setSelectedUsers(prevSelected => prevSelected.includes(userId) ? prevSelected.filter(id => id !== userId) : [...prevSelected, userId] ); }, [] // No dependencies; the function logic doesn't depend on external variables ); return ( <div> <input type="text" value={filter} onChange={e => setFilter(e.target.value)} placeholder="Search users..." /> <ul> {filteredUsers.map(user => ( <li key={user.id}> <label> <input type="checkbox" checked={selectedUsers.includes(user.id)} onChange={() => toggleUserSelection(user.id)} /> {user.name} </label> </li> ))} </ul> </div> ); }
Explanation of Example:
useEffect
for Data Fetching: We fetch user data from an API only once when the component mounts. TheuseEffect
ensures the component fetches data without causing unnecessary re-fetches.useMemo
for Filtering: We useuseMemo
to optimize filtering, recalculating the filtered list only whenusers
orfilter
changes. This avoids recomputing the filtered list on every render.useCallback
for Toggle Function: We useuseCallback
to memoize thetoggleUserSelection
function. This ensures that the function reference remains stable across renders, avoiding unnecessary re-renders of components that depend on it.
Key Takeaways
- Optimize Rendering and Recalculation: Use
useMemo
anduseCallback
to minimize unnecessary recalculations and re-renders, especially in large applications. - Side Effects and Cleanup: Utilize
useEffect
for handling side effects and cleaning up resources to prevent memory leaks. - Dependencies Matter: Be deliberate with dependencies for
useEffect
,useMemo
, anduseCallback
to ensure correct behavior and performance.
By understanding the nuances and best practices for these hooks, we can significantly enhance the efficiency and performance of our React and Next.js applications.